This lab is due by Saturday, May 27, 2023, 11:59 PM.
As with all labs you may, and are encouraged, to pair program a solution to this lab. If you choose to pair program a solution, be sure that you individually understand how to generate the correct solution.
Classes
For this lab, you will create the following Die
and Player
classes as outlined in the
following UML diagram. More details on the makeup of each class are given below. Recall that a minus symbol -
denotes a private member while a plus symbol +
denotes a public member. Be sure to follow the style
guide and naming scheme as appropriate. We want to be following the separation of private data members and public member
functions that provide access as appropriate to the underlying data members.
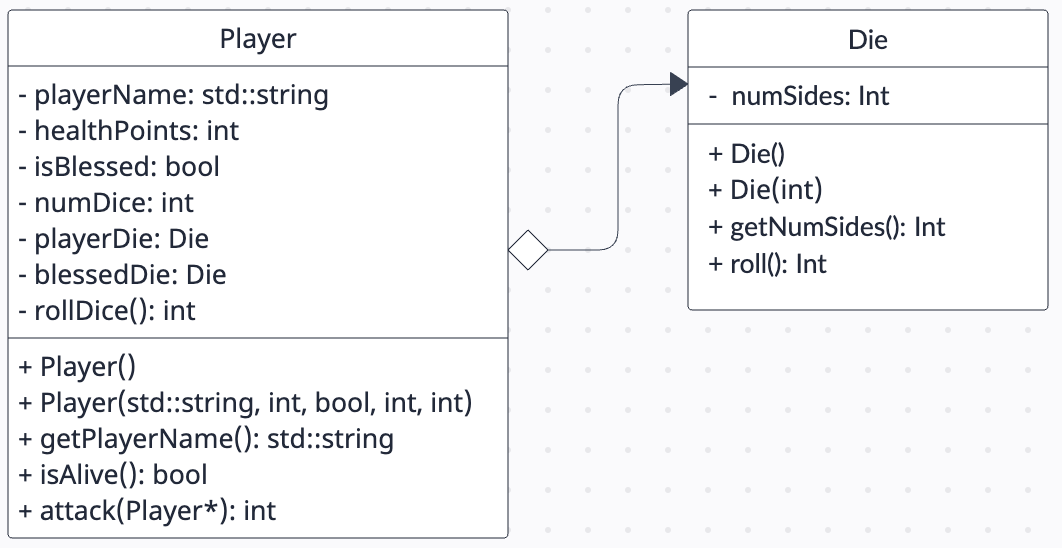
The diamond arrow in the UML diagram denotes an "association." Since the Player
class holds an instance of a
Die
object, the arrow points to the structure of the corresponding Die
class. This arrow comes
out of the side of the box to denote this dependency upon another class.
Die
Class
The Die class will be used to simulate the process of a random die roll. A description of each class member is given below:
private
members:int numSides
: data member corresponding to the number of sides on the die
public
members:Die()
: default constructor which initializes the number of sides on the die to be sixDie(int)
: parameterized constructor that validates the provided parameter is positive before assigning. If the parameter is not positive, then initializes the number of sides to be sixint getNumSides()
: returns the number of sides on the dieint roll()
: simulates a die roll by returning a random value within the range[1, numSides]
To test that your Die
class is working properly, in main()
create a D6
object for a Die
with six sides. In a loop, print the result of ten rolls. These values should all be different within the range of one to six.
Then create a D20
object for a Die
with twenty sides. In a loop, print the result of ten rolls. These values should
all be different within the range of one to twenty.
When you are satisfied with the performance of your random die, remove these testing loops from main()
and continue with the next class.
Player
Class
The Player
class will be used to represent the characters in our Samodelkin battle simulation. They will roll a number of dice
to determine their attack strength. A description of each class member is given below:
private
members:std::string playerName
: name of the playerint healthPoints
: current health of the playerbool isBlessed
: if the current player is blessed or not (blessed players sometimes deal double damage)int numDice
: the number of dice the player rollsDie playerDie
: the die the player rollsDie blessedDie
: a two sided die blessed players roll to determine if damage is doubled or notint rollDice()
: rolls theplayerDie
numDice
times and returns the sum of all dice rolls
public
members:Player()
: default constructor that initializes each data member as follows -playerName
toMadrigal
,healthPoints
to100
,isBlessed
tofalse
,numDice
to3
,playerDie
to a D6,blessedDie
to a D2Player(std::string, int, bool, int, int)
: parameterized constructor where each parameter corresponds to, in order, theplayerName
,healthPoints
,isBlessed
,numDice
, number of sides onplayerDie
. It also initializes theblessedDie
to a D2. When assigningplayerName
, if the parameter is an empty string""
then default toMadrigal
. When assigninghealthPoints
if the parameter is not positive then default to100
. When assigningnumDice
, if the parameter is not positive then default to3
.std::string getPlayerName()
: returns player's namebool isAlive()
: returnstrue
if player's current health points are greater than zero. returnsfalse
otherwiseint attack(Player*)
: first determines the sum of all the players dice - this corresponds to the damage to be dealt. Then, if the player is blessed and the blessed die rolls a2
then double the damage. Otherwise, do not modify the damage. Next, reduce the parameter's health points by the corresponding damage. Finally, return the damage dealt.
Take careful note that the parameter is a pointer to aPlayer
object. In order to access the member's off an object being pointed at, we first need to dereference the pointer and then access the member. This can be done explicitly as two operations or more simply using the arrow operator->
as shown below.
Therefore, to manipulate the parameter's health you can access it viaClass object; // object instance object.member; // access the member on the object Class *pObject = &object; // pointer to an object (*pObject).member; // dereference pointer then access the member on the object pObject->member; // dereference pointer and access the member being pointed at
pEnemy->playerHealth
(or however you appropriately named your private data member).
Steps for main()
Now in main()
, implement the following program flow to simulate a battle for the ages:
- Create a
Player
object whose name isGandalf
, has100
health, is blessed, and rolls three four-sided dice. - Create a
Player
object whose name isBalrog
, has150
health, is not blessed, and rolls two eight-sided dice. - While both players are still alive
- Have
Gandalf
attack theBalrog
- Print how much damage
Gandalf
dealt - If the
Balrog
is still alive, then- Have the
Balrog
attackGandalf
- Print how much damage the
Balrog
dealt
- Have the
- Have
- If
Gandalf
is still alive, then printGandalf has vanquished Balrog
- Else if the
Balrog
is still alive, then printBalrog has vanquished Gandalf
A sample run of the program is given below:
Gandalf attacks Balrog dealing 8 damage
Balrog counterattacks Gandalf dealing 11 damage
Gandalf attacks Balrog dealing 8 damage
Balrog counterattacks Gandalf dealing 7 damage
Gandalf attacks Balrog dealing 14 damage
Balrog counterattacks Gandalf dealing 12 damage
Gandalf attacks Balrog dealing 9 damage
Balrog counterattacks Gandalf dealing 10 damage
Gandalf attacks Balrog dealing 12 damage
Balrog counterattacks Gandalf dealing 3 damage
Gandalf attacks Balrog dealing 8 damage
Balrog counterattacks Gandalf dealing 10 damage
Gandalf attacks Balrog dealing 10 damage
Balrog counterattacks Gandalf dealing 14 damage
Gandalf attacks Balrog dealing 11 damage
Balrog counterattacks Gandalf dealing 10 damage
Gandalf attacks Balrog dealing 16 damage
Balrog counterattacks Gandalf dealing 10 damage
Gandalf attacks Balrog dealing 8 damage
Balrog counterattacks Gandalf dealing 16 damage
Balrog has vanquished Gandalf
As well as the other possible outcome:
Gandalf attacks Balrog dealing 10 damage
Balrog counterattacks Gandalf dealing 6 damage
Gandalf attacks Balrog dealing 6 damage
Balrog counterattacks Gandalf dealing 15 damage
Gandalf attacks Balrog dealing 9 damage
Balrog counterattacks Gandalf dealing 4 damage
Gandalf attacks Balrog dealing 16 damage
Balrog counterattacks Gandalf dealing 6 damage
Gandalf attacks Balrog dealing 14 damage
Balrog counterattacks Gandalf dealing 4 damage
Gandalf attacks Balrog dealing 7 damage
Balrog counterattacks Gandalf dealing 15 damage
Gandalf attacks Balrog dealing 20 damage
Balrog counterattacks Gandalf dealing 9 damage
Gandalf attacks Balrog dealing 22 damage
Balrog counterattacks Gandalf dealing 8 damage
Gandalf attacks Balrog dealing 20 damage
Balrog counterattacks Gandalf dealing 6 damage
Gandalf attacks Balrog dealing 6 damage
Balrog counterattacks Gandalf dealing 4 damage
Gandalf attacks Balrog dealing 16 damage
Balrog counterattacks Gandalf dealing 9 damage
Gandalf attacks Balrog dealing 9 damage
Gandalf has vanquished Balrog
Lab Submission
Submit your main.cpp, Die.h, Die.cpp, Player.h, Player.cpp, Makefile
file(s).
You will submit your solution to this lab with the rest of Set2. Detailed instructions for doing this are posted in Assignment 2.
This lab is due by Saturday, May 27, 2023, 11:59 PM.
As with all labs you may, and are encouraged, to pair program a solution to this lab. If you choose to pair program a solution, be sure that you individually understand how to generate the correct solution.