This assignment is due by Tuesday, April 15, 2025 by 11:59pm.
For this assignment, you will follow the same process as Samodelkin to create a new app.
When you create this project, set the package to include <userName_A3> and name the app <userName>_A3 where userName is your name (for instance, mine would be jpaone_A3).
An example of the completed app is shown below:
Part I - The Remote Data
We will use Rapid API to access the IMDB API. Create a Rapid API account and then subscribe to the Basic free level.
There will be two endpoints we connect to:
- GET autocomplete
- GET cast
When looking at the documentation for the two endpoints, the Kotlin (OkHttp) code snippet will be helpful in setting up the Retrofit request. The Example Responses will show the format that the JSON response comes in as.
Create the Kotlin classes that will mirror the structure of the JSON response. Have these classes persist to a Room database as well.
Create the appropriate Retrofit fetchr class and REST interface. Some implementation notes/recommendations:
-
It's possible to send your API Key as a query parameter. From a security standpoint, this is bad practice. Instead,
as is done with the OkHttp example, you can add it as a header to the request. Since Retrofit writes the request for us,
an Interceptor will need to be added to inject the headers into the request. There exist many articles and tutorials
for OkHttp Interceptors and Retrofit.
- When the response is received, be sure to examine the JSON body to know the relationship and structure of JSON Objects and JSON Arrays. Your POJOs will need to match this structure.
Part II - UI/UX
We'll need to make several screens for our app:
- The Movie List screen - displays a list of all the movies saved to the database
- The Search For Movie screen - search IMDB to find new movies to add to the database
- The Movie Detail screen - displays the details of a single movie and allows to search IMDB for cast members of the movie
You have freedom with how the screens exactly look, but they must meet the following requirements:
Movie List Screen
- Displays a scrollable list of all movies
- Each movie shows at a minimum the title, description, and year
- Movies can be marked as a favorite
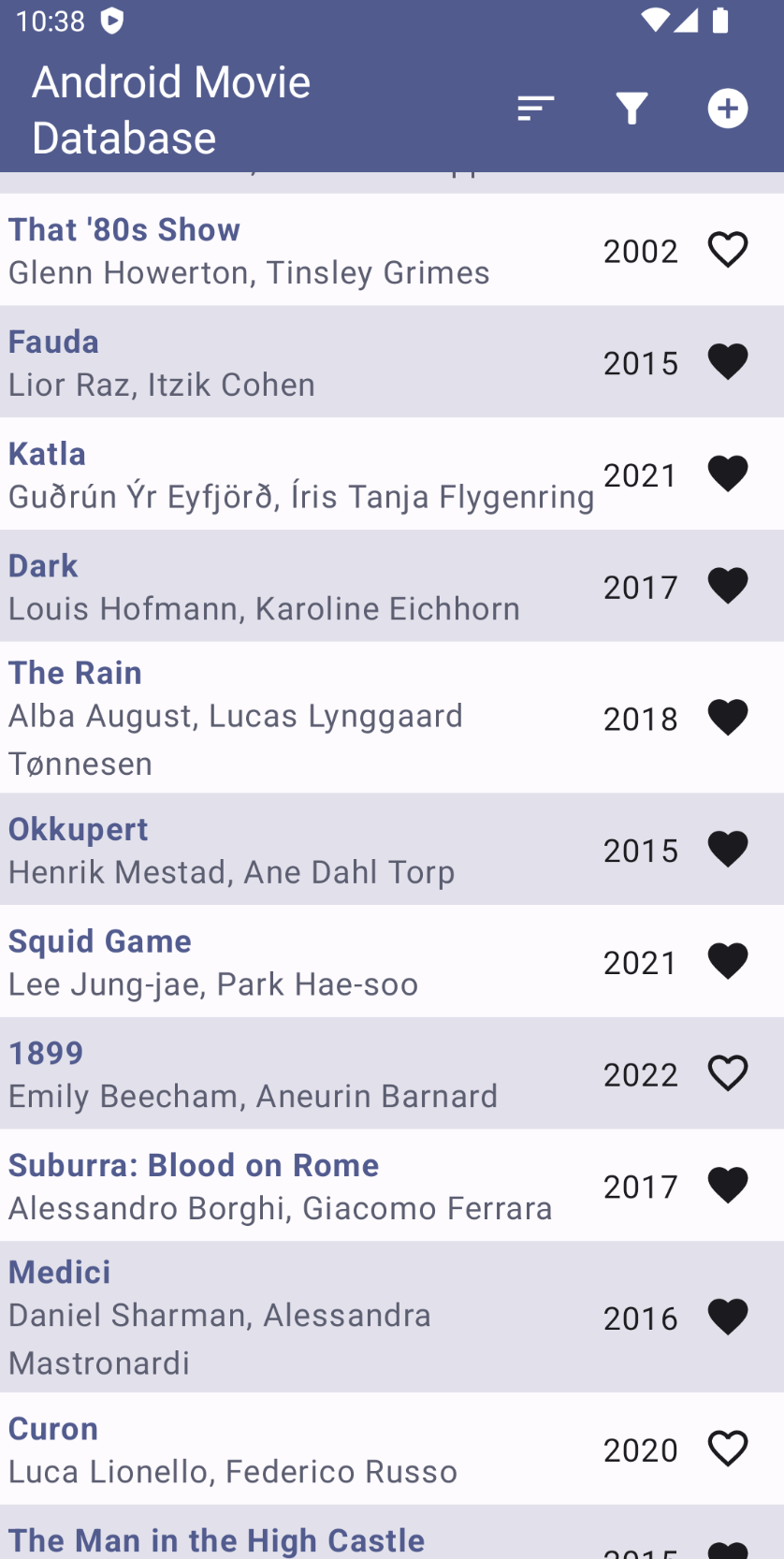
Some implementation notes/recommendations:
- The textbook can assist with a parallel example for favoriting movies and updating the database accordingly to receive an updated list appropriately.
Movie Detail Screen
- Displays the movie image, title, description, year, average rating, and genres
- Ability to search for the cast using the GET cast endpoint (only enabled if network connection exists)
- Each cast member is displayed in a scrollable list displaying the name, job, and characters
- Each cast member has a button to launch an intent to view the corresponding IMDB person webpage in application of user's choice
- Ability to delete the selected movie from the database
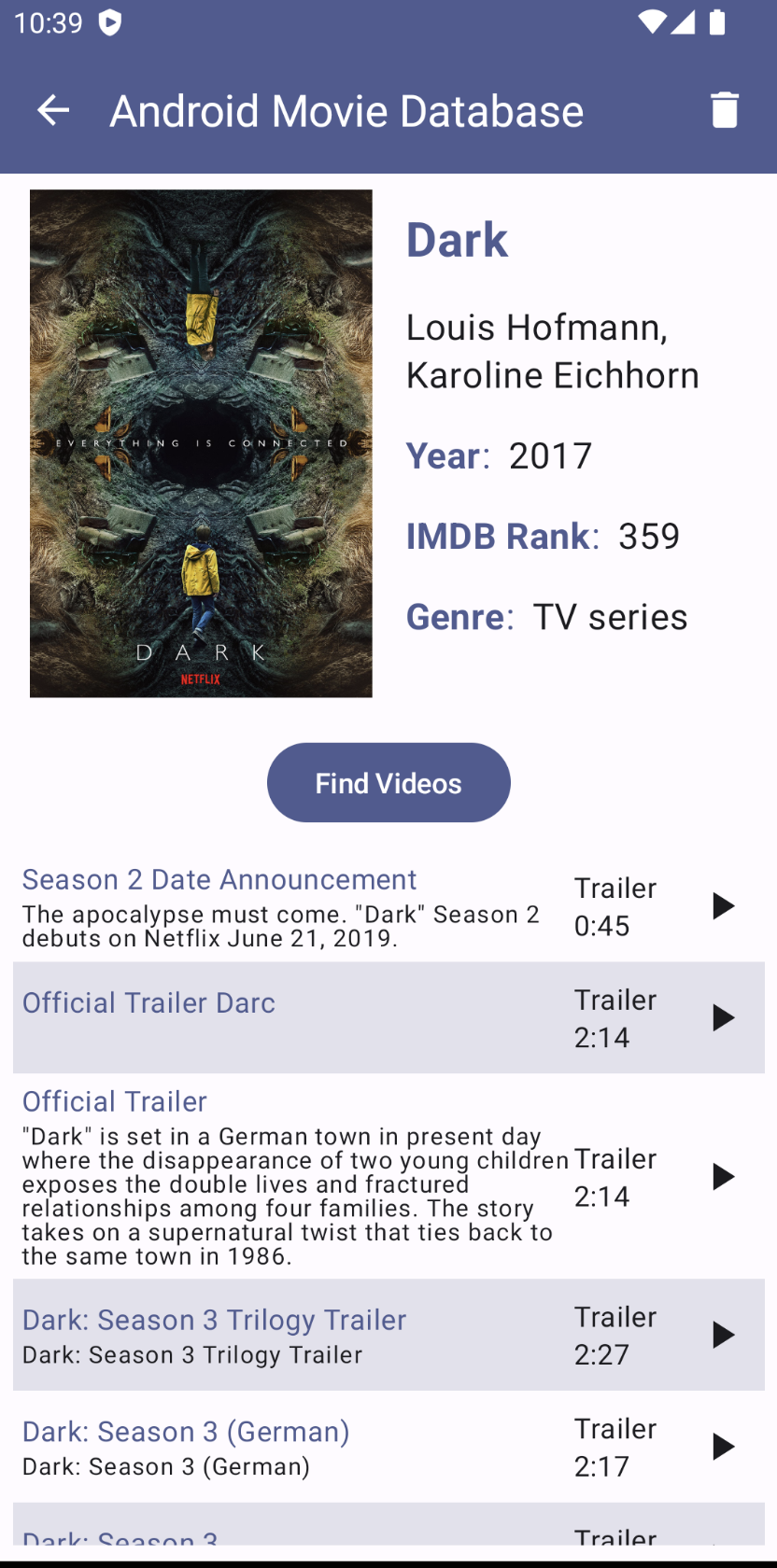
Some implementation notes/recommendations:
-
Leverage the
AsyncImage
composable function available from the COIL Library to display the remote image for the movie. See the Samodelkin app for an example of its usage. You will likely need to look at the online documentation to do a network request instead.
- When the response is received, be sure to examine the JSON body to know the relationship and structure of JSON Objects and JSON Arrays. Your POJOs will need to match this structure.
Search for Movie Screen
- Ability to search for movies using the GET autocomplete endpoint
- Button to perform search (only enabled if at least one character is entered in search field and if network connection exists)
- List of matching movies displayed in a scrollable list
- Ability to select a movie and display the associated image
- Button to save movie (only enabled if a movie is selected)
- Checks that movie to be saved does not already exist in the database
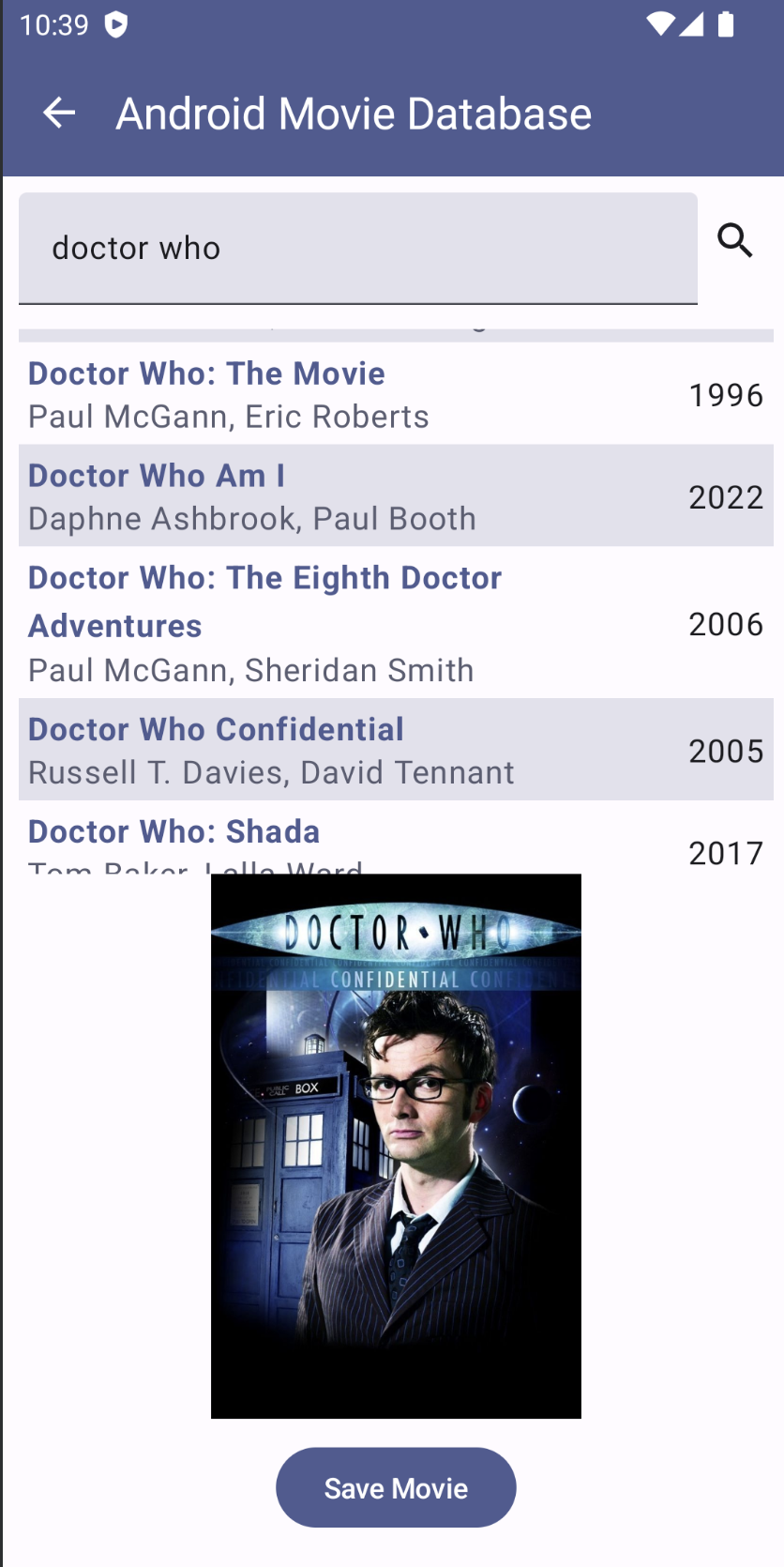
Some implementation notes/recommendations:
-
Leverage the
AsyncImage
composable function available from the COIL Library to display the remote image for the movie.
- When the response is received, be sure to examine the JSON body to know the relationship and structure of JSON Objects and JSON Arrays. Your POJOs will need to match this structure.
Part III - Navigation
We will make use of the top bar for this app.
When on the movie list screen, there will be a top bar menu item that goes to the search for movie screen. After saving a new movie, the user returns to the movie list screen and will see the movie listed. Pressing back at this point does not return to the search screen. Be sure a new destination is not added to the back stack.
Clicking on an individual movie will navigate to the movie detail screen. There will be a top bar menu item that deletes the selected movie from the database. After searching for cast, when pressing the view cast button a new app should be launched (likely a web browser) to view the cast in a webpage.
Part IV - ViewModel
Nowhere above is the ViewModel explicitly listed. You'll need to make sure it sits seamlessly between your Model and View to update both as appropriate based on data loads and user actions.
Part XC - Extra Credit
There are several opportunities for extra credit:
- Allow the user to sort the list of movies by various criteria. This is done via the top bar.
- Allow the user to filter the list of movies by various criteria. This is done via the top bar.
- When searching for a movie, once the user has entered at least three characters begin automatically searching as they continue to type.
- Give the user the opportunity to undo adding and deleting movies in the database.
See the posted video above for expected example functionality.
Documentation
With this and all future assignments, you are expected to appropriately document your code. This includes writing comments in your source code - remember that your comments should explain what a piece of code is supposed to do and why; don't just re-write what the code says in plain English. Comments serve the dual purpose of explaining your code to someone unfamiliar with it and assisting in debugging. If you know what a piece of code is supposed to be doing, you can figure out where it's going awry more easily.
Proper documentation also means
including a
README.txt
file with your submission. In your submission folder, always include a
file called
README.txt
that lists:
- Your Name / email
- Assignment Number / Project Title
- A brief, high level description of what the program is / does
- A usage section, explaining how to run the program, which keys perform which actions, etc.
- Instructions on compiling your code
- Notes about bugs, implementation details, etc. if necessary
Grading Rubric
Your submission will be graded according to the following rubric.
Will follow
Percentage | Requirement Description |
20% | Model:
|
20% | View:
|
20% | ViewModel & Utilities:
|
20% | Navigation:
|
15% | Structure:
|
5% | Submission:
|
Up to +10% | Extra Credit:
|
Submission
Please ensure your project produces an app with the name userName_A3. When you are completed with the assignment zip together your full source code (the Android Studio project), and README.txt. Name the zip file, userName_A3.zip. Upload this file to Canvas under A3.
This assignment is due by Tuesday, April 15, 2025 by 11:59pm.