This assignment is due by February 7, 2022 by 11:59pm.
For this assignment, you will make an app that calculates how many pizzas a user needs based on the hunger level of their guests. You will need to implement the necessary resources, layout, and event listeners to accomplish this task.
When you create this project, set the package to include <userName_A1> and name the app <userName>_A1 where userName is your name (for instance, mine would be jpaone_A1).
An example of the completed app is shown below:
Part I - View: Create the Layout
The full layout is shown below.
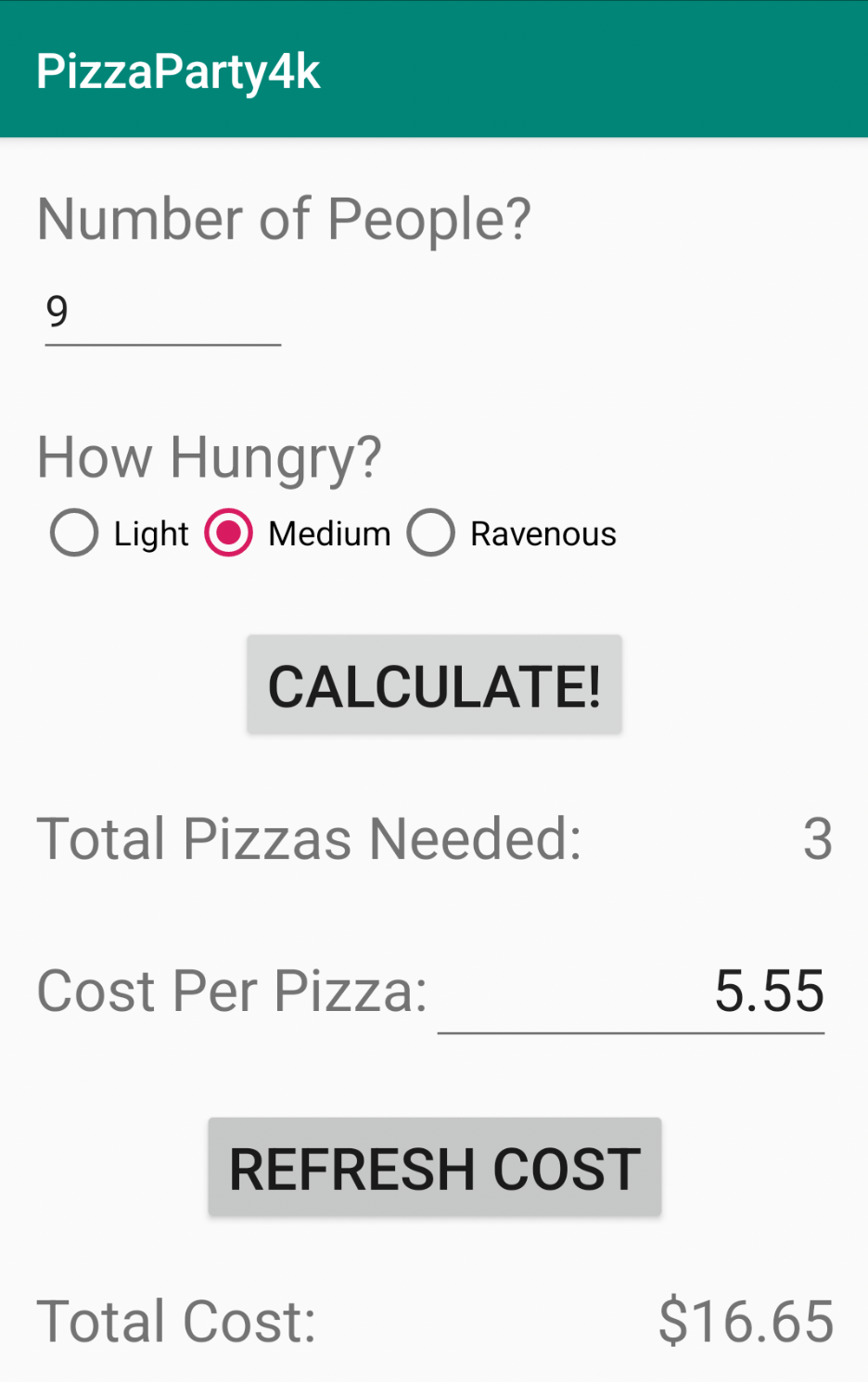
This time, there isn't a tree to traverse to build it up. You will need
to create the container and element structure by breaking the image into components.
As a hint, you'll need four LinearLayout
s, two EditText
s, a RadioGroup
of RadioButton
s, and
some other components from Lab1. How those all go together is for you to organize. You may need to do some additional research
as to how each of the View objects work.
As you are building up the layout, you'll need to make sure you are creating the appropriate string resource for each piece of text.
Part II - Activity: Add in the Logic
Next, we'll wire up our two buttons. The calculate button will take the values from the Number of People and How Hungry inputs to calculate the number of pizzas needed. The How Hungry radio buttons determine how many slices each person will eat.
- Light Hunger - 1 slice per person
- Medium Hunger - 2 slices per person
- Ravenous Hunger - 4 slices per person
Once we know the total number of slices needed for all people, we can now compute the number of pizzas needed. Each pizza
is comprised of 8 slices and we can only order whole pizzas. Thus if there are 9 medium hunger people, then 18 slices are needed
or 3 pizzas. Display the number of pizzas needed in the appropriate TextView. As with the layout piece in Part I, there may
be additional research needed to interact with the EditText
and RadioGroup
objects.
After we know the number of pizzas, we'll then calculate the total cost. By default, each pizza is $5.55. Display the cost for all the pizzas, with a $, in the appropriate TextView.
The user is able to change the price per pizza. Upon doing so, the user can then press the refresh cost button to update the total cost. There are now two triggers to update the total cost, so be sure to properly encapsulate the logic for this calculation.
Part III - View & Activity: UX/UI (or UI/UX)
When the user first opens the app, their view should be as shown below.
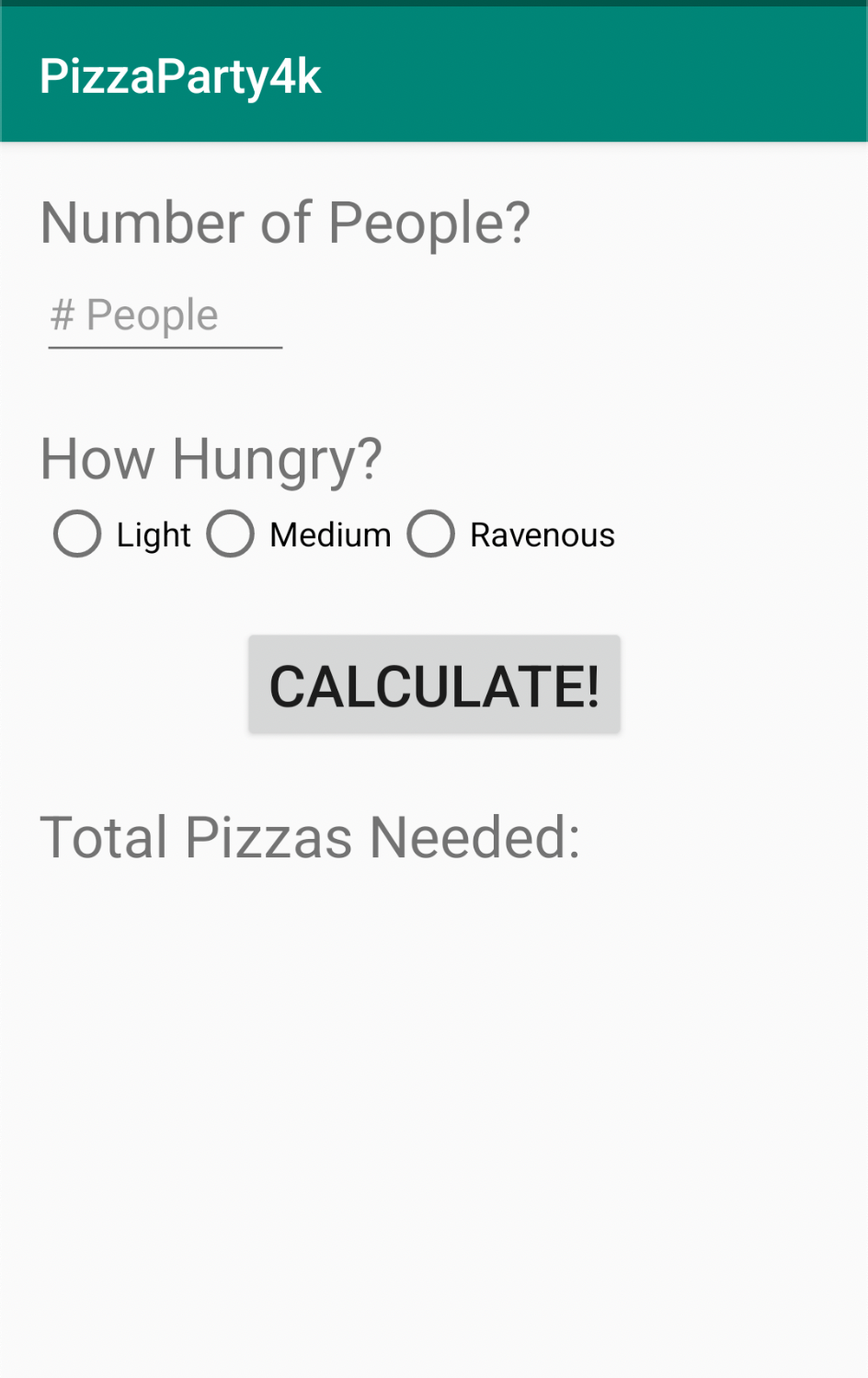
The total number of pizzas and the associated costs are initially hidden and not visible. After the calculate button is pressed for the first time, then those elements become visible. Watch the demo video above to see the expected flow. Again, some additional research will be needed to accomplish this task.
Part XC - Extra Credit
There are two extensions you can do for extra credit:
- Add in validation for our buttons. Currently, we are assuming the user will enter the number of people and hunger level before pressing the calculate button. Likewise for the cost button. Have the buttons only be enabled, and therefore clickable, after the user has entered all the required inputs. Finally, if the user clears out a field then the button should again be disabled.
- Once the total cost has been calculated via button press, have any changes to any of the inputs trigger a recalcuation.
The video below shows the extra credit in action.
Part V - Exit Interview
Be sure to include a
README.txt
file containing any comments, notes to run, etc. In addition, answer
the following questions in your
README.txt
:
- How did you handle prepending the $ symbol to the total cost?
- How did you initially hide the views and then make them visible again?
Grading Rubric
Your submission will be graded according to the following rubric.
Percentage | Requirement Description |
25% | Layout matches the provided image. |
15% | String resources used appropriately. |
15% | Event listeners implemented correctly. |
15% | UI/UX matches the first provided video. Cost is initially hidden. |
15% | App functions as expected (number of pizzas and cost calculated correctly) and structured correctly (Activity, Layout, Strings). |
5% | Exit Interview questions from Part V are answered and
included in README.txt file.
|
5% | Submission includes source code (the Android
Studio project) and README.txt .
|
5% | Submission compiles and executes without error. |
+2% | Buttons are enabled/disabled based on input. |
+3% | Changing input values triggers recalculation. |
Submission
Please update your project so it produces an executable with the name userName_A1. When you are completed with the assignment zip together your full source code (the Android Studio project), and README.txt. Name the zip file, userName_A1.zip. Upload this file to Canvas under A1.
This assignment is due by February 7, 2022 by 11:59pm.