This assignment is due by March 16, 2021 by 11:59pm.
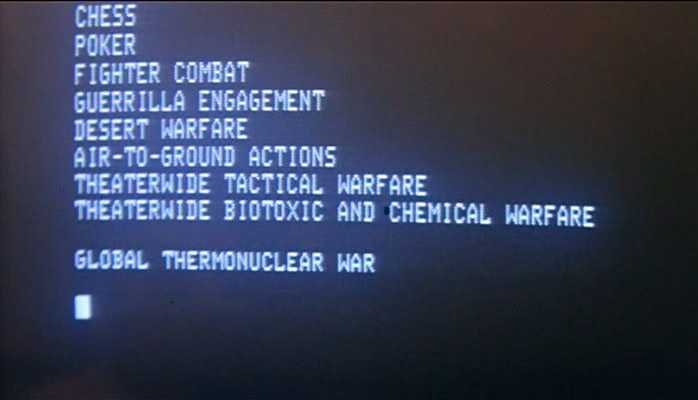
Shall we play a game?
For this assignment, you will implement a simple game such as Tic-Tac-Toe. Gameplay is not the focus of this assignment, but you must follow the technical requirements listed below.
The App will be comprised of four screens:
- Welcome
- Game
- History
- Preferences
The Welcome screen will load when the App is launched. The other three screens will be launched via menu items. Information about the game will need to be passed from fragment to fragment for the life of the App using SafeArgs and SharedPreferences as appropriate. Be sure to use a NavGraph to allow the user to navigate through the app forwards and backwards.
The App will need keep track of how many games have been won/lost/drawn and display this information to the user. These details will be stored in a database. As the user navigates between screens, the score should not reset (unless the user goes through the options, see below). Speaking of options, however the user chooses to play the game, options need to be tracked and applied to every game that is played. This is where the arguments and preferences will come into use.
When you create this project, set the package to include <userName_A2> and name the app <userName>_A2 where userName is your name.
An example of the completed app is shown below:
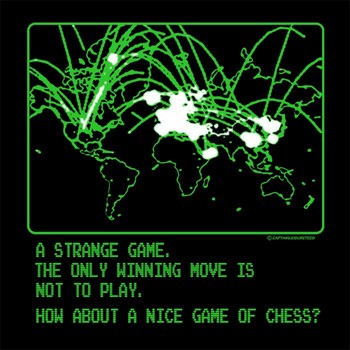
The only winning move is not to play.
Part I - Fragment One: The Welcome Screen
The layout for the Welcome Screen is very simple. Display a splash screen to serve as an intro to the game. This could be as simple as text or as complex as an image.
The menu options for this screen are:
- Play Game - brings up the Game screen
- History - brings up the History screen
- Preferences - brings up the Preferences screen
- Exit - Cleanly quits the app
Part II - Fragment Two: The Preferences Screen
The layout for the Preferences Screen will be dictated by the SharedPreferences and the corresponding Preferences XML. Present the user with options that make sense for your game. For Tic-Tac-Toe, you could allow the user to choose:
- Number of Players: 1 or 2
- AI Difficulty (only visible if 1 player chosen)
- Who Plays First? X or O
Additionally, add an option that will clear the scores and erase the database.
You may add as many options as you like (colors, themes, dark mode, special messages, X & O piece choices, etc.). Have fun with it!
The menu options for this screen are:
- None!
Part III - Fragment Three: The History Screen
You will need to keep a record of all games played in a database. The exact information stored will depend on the game you choose. One of the fields must be a Timestamp of when the game completed. For Tic-Tac-Toe, this could include:
- Winner (Player 1, Player 2, Computer)
- Piece (X, O)
- Timestamp
We'll then display all of the games in a RecyclerView. The exact layout is to your choosing, but all database information must be displayed for each record.
The menu options for this screen are:
- Play Game - brings up the Game screen
- Preferences - brings up the Preferences screen
- Exit - Cleanly quits the app
Part IV - Fragment Four: The Game Screen
This is where the magic happens. You can decide what the layout for this screen should be. This is where the actual game play will take place. Let the user(s) play the game.
When the game is over, display a Toast to the user with the outcome. Update the database with the game result. Finally, present the user with two buttons to either:
- Play Again
- Go Back
Play Again should start a new game using the same gameplay options that were previously selected. Go Back should return to the Welcome screen. These buttons will not be visible until the game is over.
With the example of Tic-Tac-Toe, the game will need some sort of 3x3 interface for the user to select where to play. Do not use simple text output for the pieces (literally "X" and "O") but instead use ImageButtons or ImageViews to spice up your game with exciting Drawables. Maybe instead of X vs O, it's the Rebels vs the Empire. You will need to use the API to find out what functions/attributes are available to you. Piazza is your friend as well. If it is set for two players, then play alternates between X and O. If gameplay is set to one player, then after the user places an X, the computer should play. The computer can be VERY SIMPLE. Have the computer select a random free space to play. No need for complex AI that never loses (though you can add it if you like - here are some strategy tips).
The menu options for this screen are:
- None!
Part V - Landscape Mode
Finally, create an appropriate landscape layout for when the screen is rotated (the game and options should all be accessible from either orientation). More importantly, you will need to save the state for every Activity/Fragment when the screen is rotated. The user should have a seamless experience. This means: (1) options do not get reset (2) the score does not get reset (3) the game does not get reset. From the user's perspective, nothing should change and there's no way to cheat at the game.
Part XC - Extra Credit
There are three extensions you can do for extra credit:
- Themes - Have one of your preferences option be a theme chooser. Selecting a new theme will change the color scheme for your app. Popular options would include light/dark mode but you can create other custom themes (NyanCat rainbows anyone?). This must be implemented via styles & themes.
- Database Filtering - When viewing the list of game history, add a menu item to filter on different criteria. For instance, show only games where Player 1/2/Computer won or show games where X always won/lost/tied, etc. This will need to return only the relevant items from the database. You should not return all items from the database and then filter in the adapter.
- Database Sorting - When viewing the list of game history, add a menu item to sort on different criteria. For instance, sort by timestamp, player name, etc. This will need to return the items from the database in the desired order. You should not return all items from the database and then sort in the adapter.
Part VI - Exit Interview
Be sure to include a
README.txt
file containing any comments, notes to run, etc. In addition, answer
the following questions in yourREADME.txt
:
- What difficulties did you encounter navigating between the various screens?
- Why did we choose to use SharedPreferences for game settings instead of placing these in the database?
Documentation
With this and all future assignments, you are expeced to appropriately
document your code. This includes writing comments in your source code
- remember that your comments should explain what a piece of code is
supposed to do and why; don't just re-write what the code says in
plain English. Comments serve the dual purpose of explaining your code
to someone unfamiliar with it and assisting in debugging. If you know
what a piece of code is supposed to be doing, you can figure out where
it's going awry more easily.
Proper documentation also means
including a
README.txt
file with your submission. In your submission folder, always include a
file called
README.txt
that lists:
- Your Name / email
- Assignment Number / Project Title
- A brief, high level description of what the program is / does
- A usage section, explaining how to run the program, which keys perform which actions, etc.
- Instructions on compiling your code
- Notes about bugs, implementation details, etc. if necessary
Grading Rubric
Your submission will be graded according to the following rubric.
Percentage | Requirement Description |
10% | WelcomeFragment classes and layout appropriately created. Appropriate landscape layout created. |
10% | SettingsFragment classes and layout appropriately created. Appropriate landscape layout created. |
10% | HistoryFragment classes and layout appropriately created. Appropriate landscape layout created. |
10% | GameFragment classes and layout appropriately created. Appropriate landscape layout created. |
15% | Proper navigation between all fragments using a NavGraph. |
10% | State is not lost during device rotation. |
10% | Game options stored via SharedPreferences. |
10% | Persistent database is used to keep game history. |
5% | Game is properly playable. Score is kept. |
5% | Exit Interview questions are answered in README.txt . |
5% | Submission includes Android
Studio project, and README.txt . Submission compiles and executes.
|
+3% | User has option to change theme of application. |
+2% | User has option to filter game results. |
+2% | User has option to sort game results. |
Submission
Please make sure your project produces an app with the name userName_A2. When you are completed with the assignment, zip together your Android Studio project (which includes the Kotlin and XML source code) and README.txt. Name the zip file, userName_A2.zip. Upload this file to Canvas under A2.
This assignment is due by March 16, 2021 by 11:59pm.