→This assignment is due by Friday, June 24, 2022, 11:59 PM.←
→ As with all assignments, this must be an individual effort and cannot be pair programmed. Any debugging assistance must follow the course collaboration policy and be cited in the comment header block for the assignment.←
→ Do not forget to complete the following labs with this set: L1A
←
· Instructions · Rubric ·Submission ·
Assignment Code Starter
Make sure you have the appropriate comment header block at the top of every assignment from this point forward. The header block should include the following information at a minimum.
/* CSCI 261: Assignment 1: A1 - Rock Paper Scissor Throw Down!
*
* Author: XXXX (INSERT_NAME)
* Resources used (Office Hours, Tutoring, Other Students, etc & in what capacity):
* // list here any outside assistance you used/received while following the
* // CS@Mines Collaboration Policy and the Mines Academic Code of Honor
*
* XXXXXXXX (MORE_COMPLETE_DESCRIPTION_HERE)
*/
// The include section adds extra definitions from the C++ standard library.
#include <iostream> // For cin, cout, etc.
// We will (most of the time) use the standard library namespace in our programs.
using namespace std;
// Define any constants below this comment.
// Must have a function named "main", which is the starting point of a C++ program.
int main() {
/******** INSERT YOUR CODE BELOW HERE ********/
cout << "Hello world!" << endl; // print Hello world! to the screen
/******** INSERT YOUR CODE ABOVE HERE ********/
return 0; // signals the operating system that our program ended OK.
}
Rock Paper Scissors
Most of you have likely played the classic game Rock, Paper, Scissors. Believe it or not, a hardcore world of Rock, Paper, Scissors has existed, even in Denver. It's a bit frightening.... So, before you start thinking how Rock, Papers, Scissors (or RPS for those in the know) is a kid's game, think again. This is serious stuff; go ahead and TRY to beat the computer on this NY Times link. (But, if anyone asks, your C++ assignment this week is to implement a simple interactive rule-based system that addresses a theoretical Decision Problem.)
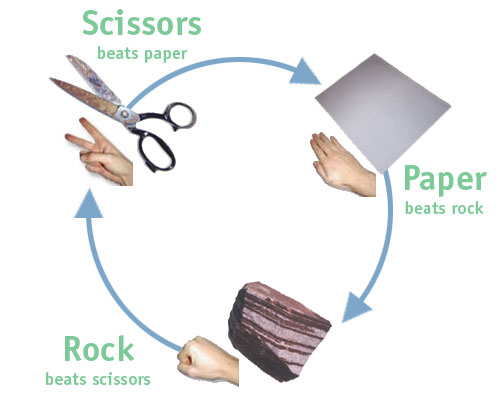
Your goal is to ultimately implement a one-player version of Rock, Paper, Scissors against the computer.
The User's Choice
The first step is to ask the Human Player what they choose
and then repeat back their choice.
The Human Player can enter either R
or r
for rock, P
or p
for paper, and S
or s
for scissors. Instead of printing
back whatever the Human entered, we want to display the full word that corresponds to the single letter entered. Here is an example interaction for this part of the program:
Welcome one and all to a round of Rock, Paper, Scissors! (Enter P, R or S)
Player: R
Player choose Rock
Here is another example:
Welcome one and all to a round of Rock, Paper, Scissors! (Enter P, R or S)
Player: p
Player choose Paper
Note: We will assume a smart user that follows directions and will enter a valid value.
The Computer's Choice
Now, we must randomly decide what the Computer chooses. To do so,
we will randomly generate a number for the computer. The computer has three possible choices and we
will represent these three choices by the numbers 0, 1, and 2. Properly use the rand()
function to generate a random number in the range [0, 2]. Instead
of displaying 0, 1, or 2, your program should instead print Rock, Paper, or Scissors respectively. At
this point, we now will know what each player has thrown:
Welcome one and all to a round of Rock, Paper, Scissors! (Enter P, R or S)
Player one: S
Player choose Scissors
Computer choose Scissors
Welcome one and all to a round of Rock, Paper, Scissors! (Enter P, R or S)
Player one: S
Player choose Scissors
Computer choose Rock
Welcome one and all to a round of Rock, Paper, Scissors! (Enter P, R or S)
Player one: S
Player choose Scissors
Computer choose Paper
Every time you run your program, the computer should have a different value. Once your random number is working properly, it's time to make sense of both players' choices and move on.
Declare a Winner
The final step of our game is to determine who actually won. The handy chart above will be your guide.
Add a final line of output that prints a line following this pattern:
X beats Y. Z wins!
Where X and Y are one of "rock" or "scissors" or "paper" and Z is either "Human" or "Computer". Be sure to handle ties appropriately too. And now, we have a fully functioning Rock, Paper, Scissors game! Great job!
Welcome one and all to a round of Rock, Paper, Scissors! (Enter P, R or S)
Player one: R
Player choose Rock
Computer choose Paper
Paper beats Rock.
Computer wins!
Let's Play Two!
Now we want to modify our game so the user can continue to play another game if they choose.
As the user continues to play, keep track of how many games the user won, lost, and tied. When the user stops playing, print out a nice message and how many games were won, lost, and tied.
A sample run of the program is shown below:
Welcome one and all to a round of Rock, Paper, Scissors! (Enter P, R or S)
Player one: R
Player choose Rock
Computer choose Paper
Paper beats rock.
Computer wins!
Do you want to play again? (Y/N) Y
Welcome one and all to a round of Rock, Paper, Scissors! (Enter P, R or S)
Player one: R
Player choose Rock
Computer choose Paper
Paper beats rock.
Computer wins!
Do you want to play again? (Y/N) Y
Welcome one and all to a round of Rock, Paper, Scissors! (Enter P, R or S)
Player one: R
Player choose Rock
Computer choose Scissors
Rock beats scissors.
Player wins!
Do you want to play again? (Y/N) N
Thanks for playing!
You won 1 game(s), lost 2 game(s), and tied 0 game(s).
Congrats! We've now fully finished our Rock, Paper, Scissors game. See how many games you can play in a row without losing to the computer!
Grading Rubric
Your submission will be graded according to the following rubric.
Points | Requirement Description |
4 | All code submitted properly. |
5 | All labs completed and submitted. |
6 | User can input values. |
3 | Computer generates correct values. |
9 | Correct game outcome determined. |
6 | Multiple games can be played. |
3 | Game stats tracked. |
3 | I/O and program flow matches examples. |
4 | (1) Comments used. (2) Coding style followed. (3) Appropriate variable names, constants, and data types used. (4) Instructions followed. |
43 | Total Points |
→This assignment is due by Friday, June 24, 2022, 11:59 PM.←
→ As with all assignments, this must be an individual effort and cannot be pair programmed. Any debugging assistance must follow the course collaboration policy and be cited in the comment header block for the assignment.←
→ Do not forget to complete the following labs with this set: L1A
←
Submission
Always, always, ALWAYS update the header comments at the top of your main.cpp file. And if you ever get stuck, remember that there is LOTS of help available.
It is critical that you follow these steps when submitting homework.
If you do not follow these instructions, your assignment will receive a major deduction. Why all the fuss? Because we have several hundred of these assignments to grade, and we use computer tools to automate as much of the process as possible. If you deviate from these instructions, our grading tools will not work.
Submission Instructions
Here are step-by-step instructions for submitting your homework properly:
-
Make sure you have the appropriate comment header block at the top of every source code file for this set. The header
block should include the following information at a minimum.
Be sure to fill in the appropriate information, including:/* CSCI 261: Assignment 1: A1 - Rock Paper Scissor Throw Down!
* * Author: XXXX (INSERT_NAME) * Resources used (Office Hours, Tutoring, Other Students, etc & in what capacity): * // list here any outside assistance you used/received while following the * // CS@Mines Collaboration Policy and the Mines Academic Code of Honor * * XXXXXXXX (MORE_COMPLETE_DESCRIPTION_HERE) */- Assignment number
- Assignment title
- Your name
- If you received any type of assistance (office hours - whose, tutoring - when), then list where/what/who gave you the assistance and describe the assistance received
- A description of the assignment task and what the code in this file accomplishes.
Additionally, update theMakefile
for A1 to generate a target executable namedA1
.
- File and folder names are extremely important in this process.
Please double-check carefully, to ensure things are named correctly.
- The top-level folder of your project must be named
Set1
- Inside
Set1
, create 2 sub-folders that are required for this Set. The name of each sub-folder is defined in that Set (e.g.L1A
, andA1
). - Copy your files into the subdirectories of
Set1
(steps 2-3), zip thisSet1
folder (steps 4-5), and then submit the zipped file (steps 6-11) to Canvas. - For example, when you zip/submit
Set1
, there will be 2 sub-folders calledL1A
, andA1
inside theSet1
folder, and each of these sub-folders will have the associated files.
- The top-level folder of your project must be named
- Using Windows Explorer (not to be confused with Internet Explorer), find the files
named
main.cpp, Makefile
.
STOP: Are you really sure you are viewing the correct assignment's folder? - Now, for A1, right click on
main.cpp, Makefile
to copy the files. Then, return to theSet1/A1
folder and right click to paste the files. In other words, put a copy of your homework'smain.cpp, Makefile
source code into theSet1/A1
folder.
Follow the same steps for each lab to put a copy of each lab's deliverable into theSet1/L1
folders. Do this process forSet1/L1A
(main.cpp
).
STOP: Are you sure yourSet1
folder now has all your code to submit?
- Now, right-click on the
"Set1"
folder.- In the pop-up menu that opens, move the mouse
"Send to..."
and expand the sub-menu. - In the sub-menu that opens, select
"Compressed (zipped) folder"
.
STOP: Are you really sure you are zipping aSet1
folder with sub-folders that each contain amain.cpp
file in it?
- In the pop-up menu that opens, move the mouse
- After the previous step, you should now see a
"Set1.zip"
file.
- Now visit the Canvas page for this course
and click the
"Assignments"
button in the sidebar.
- Find Set1, click on it, find the
"Submit Assignment"
area, and then click the"Choose File"
button.
- Find the
"Set1.zip"
file created earlier and click the"Open"
button.
STOP: Are you really sure you are selecting the right homework assignment? Are you double-sure?
- WAIT! There's one more super-important step. Click on the blue
"Submit Assignment"
button to submit your homework.
- No, really, make sure you click the
"Submit Assignment"
button to actually submit your homework. Clicking the"Choose File"
button in the previous step kind of makes it feel like you're done, but you must click the Submit button as well! And you must allow the file time to upload before you turn off your computer!
- Canvas should say "Submitted!". Click "Submission Details" and you can download the zip file you just submitted. In other words, verify you submitted what you think you submitted!
In summary, you must zip the "Set1"
folder
and only the "Set1"
folder, this zip folder must have several sub-folders, you must name all these folders correctly, you must submit the correct zip file for this
homework, and you must click the "Submit Assignment"
button. Not doing these steps is like bringing your
homework to class but forgetting to hand it in. No concessions will be made for
incorrectly submitted work. If you incorrectly submit your homework, we will not be able to
give you full credit. And that makes us unhappy.
→This assignment is due by Friday, June 24, 2022, 11:59 PM.←
→ As with all assignments, this must be an individual effort and cannot be pair programmed. Any debugging assistance must follow the course collaboration policy and be cited in the comment header block for the assignment.←
→ Do not forget to complete the following labs with this set: L1A
←