Python Lab 8: Vowels or Not?
Due Tuesday, October 26th, by 11:45pm
Introduction
To get started, open IDLE and then create a New File via the File menu.
We suggest you immediately save this file in the directory you created to manage all your
Python Labs this semester (e.g., CSCI101/PythonLabs). Save this file as
Lab8-string_game.py.
Introduction
Kevin and Stacy want to play a game they have
titled 'Vowels or Not?'. Here are the game rules:
- Both players are given the same string, S.
- Both players have to make substrings using the letters of the
string S.
- Kevin has to make substrings starting with consonants.
- Stacy has to make substrings starting with vowels. (Note: vowels are only
defined as AEIOU, i.e., in this game, Y is not considered a vowel.)
- The game ends when both players have made all possible substrings.
A player gets +1 point for each occurrence of the substring in the string
S. For example, consider the string S = BANANA.
Suppose Stacy's vowel beginning substring is ANA. In this case,
ANA occurs twice in BANANA. Hence, Stacy will get 2 points
for this substring. See the following figure for other examples. Your task is
to determine the winner of the game and their score, given a string S.
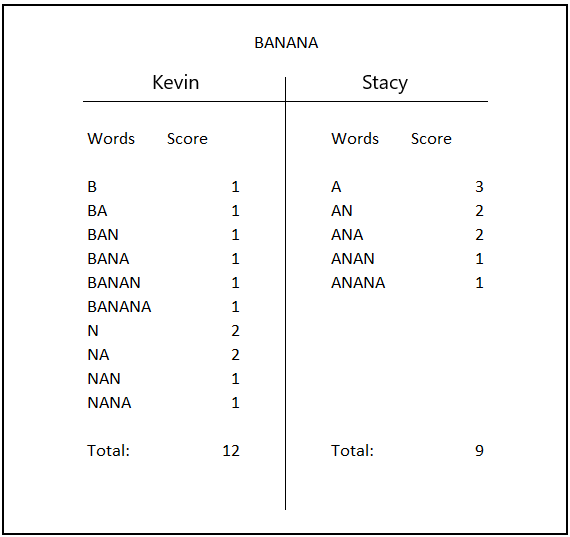
Your program should:
- Ask the user whether they want to provide a string for the game (choice 1)
OR whether they prefer to have a randomly generated string (choice 2).
- If the user enters 1, ask the user to enter a string S. The user
should enter a string of all uppercase letters.
- If the user enters 2, ask the user for a seed and then generate a random
string of 6 uppercase letters. Random should be seeded as a string.
You must generate the random string by creating a list/string of all 26
uppercase letters and then using random.choice() to choose 6 random characters.
- Output Kevin's score for the game, followed by Stacy's score for the game.
- Output the winner of the game (Kevin or Stacy) or state the game was a
"Draw" (if their scores are the same).
Hint1: there are a couple different ways that you could generate a string of random letters. See the end of
Python Video #14: RNG
for one idea. Other ways also exist using, for example, the string constant string.ascii_uppercase.
Hint2: Example code to request/use a seed is shown next.
my_seed = input("Seed to initialize the random generator: ")
random.seed( my_seed )
Lab I/O Format
Throughout this semester we use a specific Lab Input/Output Format.
This format is described below:
- When prompting for input, use the prompt string
WORD> , where
WORD is a single, uppercase word which
describes the input. For example, your WORD s
in this lab might be: CHOICE> ,
STRING> and
SEED>
- When providing output that will be graded, start the line with
OUTPUT . Think of this as "boxing your
answer" on a math worksheet; it lets us quickly find your
answer. Our grading script will skip any output lines that do
not start with OUTPUT .
- You are welcome (and should!) have other output lines that do
not begin with OUTPUT; while our grading
script will ignore these, good programmers include print
statements that are informational to the user of the program.
- A submission without exactly correct output formatting will receive an
AUTOMATIC ZERO. This is because Gradescope is automated - it does not
look at your code, only the results, and thus the format of the results must
be consistent for all students.
Example Execution 1:
Would you like to provide your own string or generate a random one? (1 or 2)
CHOICE> 1
Enter a string for the game:
STRING> BANANA
With the string BANANA, Kevin's score is 12 and Stacy's score is 9
Kevin wins!
OUTPUT 12
OUTPUT 9
OUTPUT Kevin
Example Execution 2:
Would you like to provide your own string or generate a random one? (1 or 2)
CHOICE> 2
Number to initialize the random generator:
SEED> 123456789
The randomly generated string for this game is FIFQZM:
With the string FIFQZM, Kevin's score is 16 and Stacy's score is 5
Kevin wins!
OUTPUT 16
OUTPUT 5
OUTPUT Kevin
Example Execution 3:
Would you like to provide your own string or generate a random one? (1 or 2)
CHOICE> 1
Enter a string for the game:
STRING> AND
With the string AND, Kevin's score is 3 and Stacy's score is 3
It's a tie!
OUTPUT 3
OUTPUT 3
OUTPUT Draw
Gradescope Submission Nuances
When you submit your Python file to Gradescope, multiple different test cases
are run on your code. Passing all of the tests results in a 100% on the autograded
portion of the lab.
You are allowed to submit to Gradescope four times (or less) for this lab.
Please IGNORE the automated email from Gradescope, this information is incorrect. The maximum grade of your submissions will be your grade for the lab. Note: If
your code doesn't work (e.g., a syntax error exists, or an error is thrown in
execution), then you will receive an AUTOMATIC ZERO. You should test your
code before submitting to ensure it executes correctly.
Comments
All Python files should include a header with your name,
section, assignment info, references (i.e., who did you collaborate
with on this assignment?; what resource did you use?), and approximate
time taken to do the assignment. Be sure to cite any
allowed external references used to complete the assignment.
Any code without this header will lose 1 point. Here's an
example:
# Tracy Camp
# CSCI 101 - Section F
# Python Lab 8
# References: web site for generating pseudo-random numbers
# References: https://docs.python.org/3/library/random.html
# Time: 45 minutes
Submission
Once you are satisfied with your solution to this lab, you need to submit the file to
Gradescope. In Gradescope, go to CSCI 101 > Lab8 and upload Lab8-string_game.py.
Note: this lab is worth 6 points. To receive full credit, your
code must execute in Python 3, and you must
submit a single file (your Python code file). In addition, your
input/output must match the lab requirements.
Whenever you submit something to Gradescope, we strongly recommend you always double check
what you submitted actually got submitted correctly (e.g., did the file upload correctly?
did you submit the correct file? etc.) If your submission is incorrect, it's on you.
|